SpringCloud 微服务构建一:服务的注册与发现-Eureka
一:服务发现
在微服务架构中,服务发现组件是很关键的一个组件,服务发现组件就是去管理各服务的网络地址等信息。
服务提供者、服务消费者、服务发现组件的关系:
- 服务启动时,会将自己的网络地址等信息注册到服务发现组件中,让服务发现组件去存储管理这些信息。
- 服务消费者从服务发现组件这里查询服务提供者的网路地址信息,并使用该地址去调用服务提供者的接口。
- 各服务和服务发现组件通过一定的机制通信(心跳)。
Spring Cloud 支持多种服务发现组件,如 Eureka、Consul 和 Zookeeper 等,这里主要介绍 Spring Cloud Eureka 的使用。
二:Eureka 介绍
Eureka 是 Netflix 开源的服务治理模块,本身是一个基于 Rest 的服务。
- 基于 Netflix Eureka 做了二次封装
- 两个组件组成:
- Eureka Server 注册中心
- Eureka Server 提供服务发现的功能,服务启动后,向 Eureka Server 注册自己的地址信息(IP、端口、服务名)
- 当 Eureka Server 在一定时间内接收不到某个服务实例的心跳,将会注销该实例,默认时间为 90 秒
- 默认情况下 Eureka Server 同时也是 Eureka Client。多个 Eureka Server 实例通过复制的方式来实现服务注册
- Eureka Client 服务中心
- Eureka Client 是一个客户端,用于与 Eureka 的交互 0 服务启动后,与 Eureka Server 通过 心跳 机制通信,默认周期为 30 秒
- Eureka Client 有 缓存机制,会缓存服务注册表中的信息。服务不需要每次请求都查询 Eureka Server,这样既降低了 Server 的压力,同时即便 Eureka Server 的节点都宕机了,也可以使用缓存查询到服务提供者的信息完成调用
Eureka 架构图:
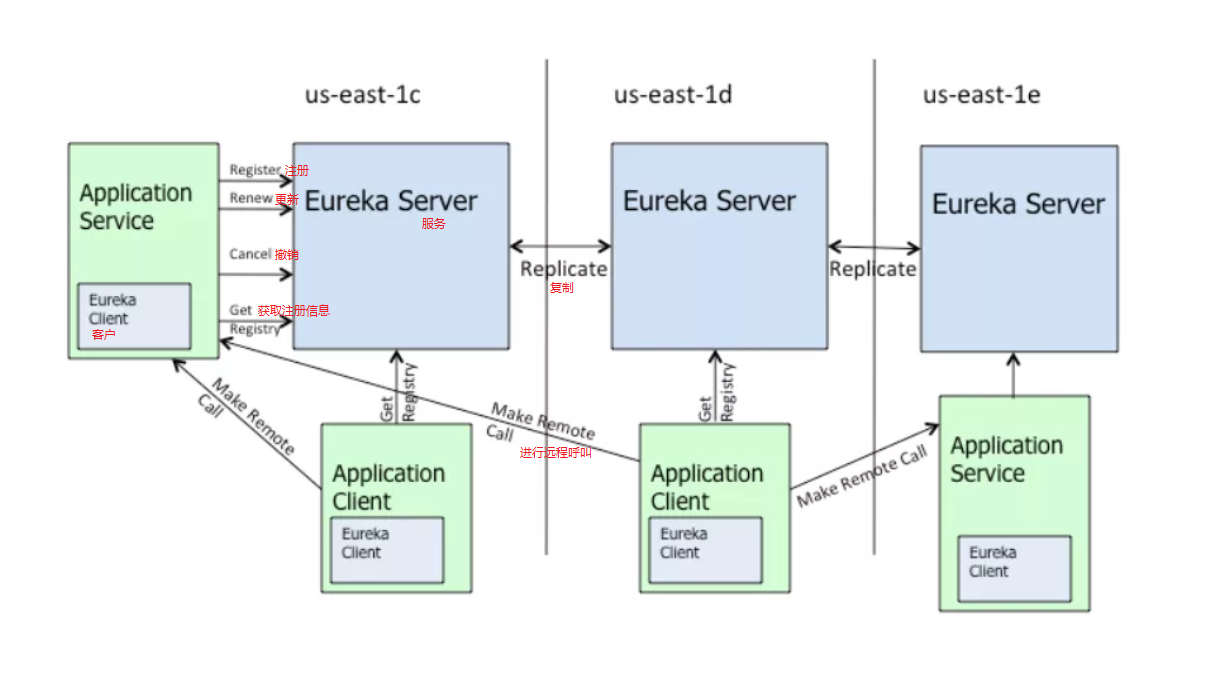
Eureka
eureka server –注册中心服务端
创建一个 maven 项目,之后在 pom 文件添加 Eureka Server 的依赖,pom.xml 主要内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.9.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>eureka</artifactId> <version>0.0.1-SNAPSHOT</version> <name>eureka</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> <spring-cloud.version>Greenwich.SR3</spring-cloud.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
|
创建一个启动类:EurekaApplication
启动前在 EurekaApplication 启动类加上 Eureka 服务的注解:
1 2 3 4
| @SpringBootApplication@EnableEurekaServer public class EurekaApplication { public static void main(String[] args) { SpringApplication.run(EurekaApplication.class, args); }}
|
启动之后可以打开 127.0.0.1:8080(默认为 8080)
进入注册中心,则 Eureka Server 算初步完成创建
启动之后你会发现控制台在报错:

因为 Server 也是 Client,所以自己也需要注册进服务里面,有两种方式解决:
- 将自己注册进自己的服务里
- 配置自己不注册到服务中
二选一就能够解决控制台的报错!
配置文件:
1 2 3 4 5 6 7 8 9 10 11 12
| spring: application: name: eureka-server1 server: port: 8761 eureka: client: service-url: defaultZone: http://127.0.0.1:8761/eureka/ 、
|
配置完之后重启服务,报错消失了!
由于还没有服务注册,所以没有被发现的服务:
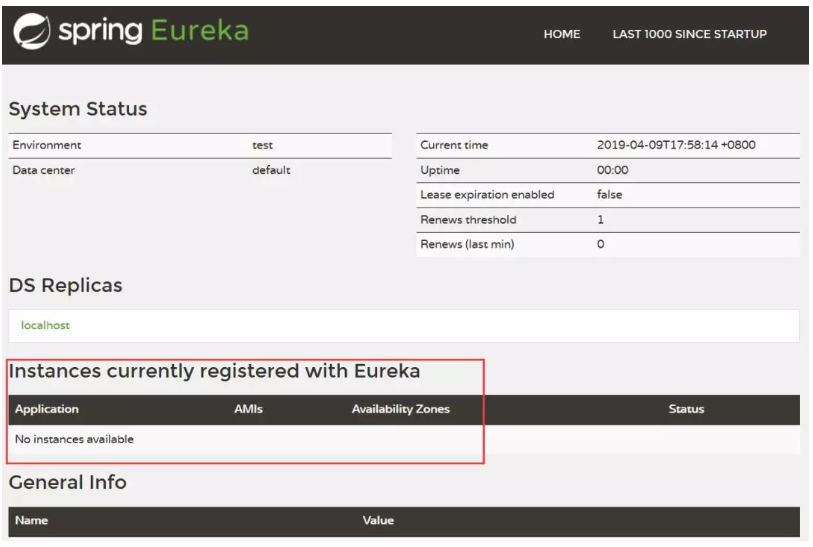
eureka client –注册服务客户端
创建完注册中心后,创建服务客户端
新建一个 maven 项目,在 pom.xml 添加 Eureka Client 依赖:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.9.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>client</artifactId> <version>0.0.1-SNAPSHOT</version> <name>client</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> <spring-cloud.version>Greenwich.SR3</spring-cloud.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build>
|
同样的要先创建一个启动类 ClientApplication
启动前也要在 ClientApplication 启动类上添加注解:
1 2 3 4 5 6 7
| @SpringBootApplication
public class ClientApplication { public static void main(String[] args) { SpringApplication.run(ClientApplication.class, args); }}
|
客户端不同注册中心能之间启动,需要先配置
1 2 3 4 5 6 7 8 9
| server: port: 7761 spring: application: name: client-server eureka: client: service-url: defaultZone: http://127.0.0.1:8761/eureka/
|
先启动好 Eureka Server,再启动 Eureka Client
都启动之后,在你的注册中心有你的 client 注册进去,则 Eureka Client 算初步完成创建

Eureka 总结:
- @EnableEurekaServer @EnableEurekaClient
- 心跳检查、健康检查、负载均衡等功能
- Eureka 的高可用,生成上建议至少两台以上
- 分布式系统中,服务注册中心是最重要的基础部分
鸣谢: